Today at 8 AM there will be a MANDATORY (if you have not finished the Matrix problem) Google Hangout where I will review 2D arrays and go over the matrix problem. To join the Google Hangout be sure you login to your google account first then click on this link - meet.google.com/pfc-oyxm-and
Have two windows open, one with the hangout window and the other with Codiva or Eclipse so you can write your own code during the presentation. Don't be afraid to ask questions during the presentation.
If you missed today's hangout session email me with any questions you may have or setup your own hangout session and invite me to it. Email me first to arrange a time for the hangout session.
Review Two Dimensional Arrays and ArrayPractice2
Today we start on Step 1 of the Matrix Problem:
In Algebra class we learn about matrices. We learn to add, subtract, and multiply 2x2 matrices and 3x3 matrices. Below are some examples from Algebra class with the answers:
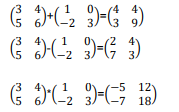
It is almost apparent how to add. We add the first position in the first matrix with the first position in the second matrix. We continue with the corresponding positions to get the answer. Subtraction follows this same positional methods. Multiplication of matrices appears to be confusing since it does not follow the positional method used in addition and subtraction. The answer is achieved by taking the row from the first matrix and the column from the second matrix and multiplying the respective values and then taking the sum of the products. The answer above was achieve as follows:
3(1)+4(-2)=-5 3(0)+4(3)=12 5(1)+6(-2)=-7 5(0)+6(3)=18
Write a program that take in two matrices and then allow the user to choose to add, subtract, or multiply them and display the answer. The program will display the following menu:
1. Enter Matrix A
2. Enter Matrix B
3. Display A + B
4. Display A - B
5. Display A * B
6. Exit
The program should loop and allow the user to continue to choose different options until they choose quit. The well written program will modularize the process into different methods.
Since this is a complex problem we will break it down into smaller tasks. Here are the tasks you need to complete:
Step 1. Create your variables, one for the users choice, three for the arrays you will use call the first array array1, the second array2, and the third array3. Array 3 is the array you will store your calculated values in. The arrays are two dimensional arrays with two rows and two columns. You will also need a Scanner object to allow the user to enter their choice as well as the number for the array, call this input.
Step 2. Create a loop that displays the menu and continues to execute until the user chooses to exit.
What type of loop will you use for this?
For each option at this point just print out the option they chose, so if the user enters 1 the program would simply print out Enter Matrix A but it would not do anything else. Later you will add the code to perform this task.
Here is what your output should look like:
Welcome to the Matrix Calculator. Please choose one of the following options:
1. Enter Matrix A
2. Enter Matrix B
3. Display A + B
4. Display A - B
5. Display A * B
6. Exit
1
Enter Matrix A
4
Display A - B
6
Exit
Thank you for using the Matrix Calculator. This program was brought to you by ... . Have a nice day.
Due Today! - Create this loop and run it in Codiva. Copy the code and then take a screen shot of the output. Submit to Canvas for Matrix1 Menu.
Step 3. Create a printArray( ) method that accepts any 2D array of integers and prints out the array for the user. Remember that you declare methods outside of your main method so find the end of your main method } and then write your method after the closing } of the main method.
Here is the start of the code, you will need to fill in everything between the { }
public static void printArray(int[][] array) {
You will need a for loop that iterates through the 2D array, refer to the example in ArrayPractice2.
Just a hint here, in the outer loop you will use
just use println() to force a line return.
In the inner loop you will use print( ) to print each array value and a space.
Since the parameter
that you are passing to this method is array this is the array you will print.
}
Test your method by calling it in your main method and using array1 as the parameter
printArray(array1);
Since you have not provided any values to array1 the output should look like this:
0 0
0 0
Run your code in Codiva. Copy the code and then take a screen shot of the output. Submit to Canvas for Matrix2 Print Array.
Hints for the Matrix program Part 2 - Filling the Array