Review Two Dimensional Arrays and ArrayPractice2
Here is the Matrix Problem:
In Algebra class we learn about matrices. We learn to add, subtract, and multiply 2x2 matrices and 3x3 matrices. Below are some examples from Algebra class with the answers:
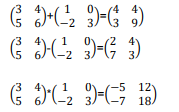
It is almost apparent how to add. We add the first position in the first matrix with the first position in the second matrix. We continue with the corresponding positions to get the answer. Subtraction follows this same positional methods. Multiplication of matrices appears to be confusing since it does not follow the positional method used in addition and subtraction. The answer is achieved by taking the row from the first matrix and the column from the second matrix and multiplying the respective values and then taking the sum of the products. The answer above was achieve as follows:
3(1)+4(-2)=-5 3(0)+4(3)=12 5(1)+6(-2)=-7 5(0)+6(3)=18
Write a program that take in two matrices and then allow the user to choose to add, subtract, or multiply them and display the answer. The program will display the following menu:
1. Enter Matrix A
2. Enter Matrix B
3. Display A + B
4. Display A - B
5. Display A * B
6. Exit
The program should loop and allow the user to continue to choose different options until they choose quit. The well written program will modularize the process into different methods.
Since this is a complex problem we will break it down into smaller tasks. Here are the tasks you need to complete:
At this point you should have a working menu and a printArray( ) method.
Step 4. Create a fillArray( ) method that prompts the user four times to enter a value and each time a value is entered places it in the array. This method will have two parameters, a Scanner object input that accepts the users input value and then the array that you want the values to be place in.
fillArray(Scanner input, int[][] array) {
Again you will need a for loop that iterates through the 2D array, refer to the example in ArrayPractice2.
Just a hint here, you can copy and paste the loop you used in the printArray( ) method and then remove the System.out.print statements and replace them with your new code.
Insid the inner loop you will need to prompt the user for a value for the matrix.
Assign that value to the array.
Outside of the for loop you will want to call the printArray( ) method so the user can see the matrix they entered.
}
Test your method by calling it in your menu. After the user enters the values the array should print.
At this point menu items 1 and 2 should work.
When choice 1 is chosen and the user enters the values of 3, 4, 5 and 6 the output should look like this:
3 4
5 6
Run your code in Codiva. Copy the code and then take a screen shot of the output. Submit to Canvas for Matrix Step 4.
Move onto Matrix Part 3